Magento 2: Creating a custom theme
Photo by Maria Eklind, CC BY-SA 2.0
In my previous post, we went through the steps needed to create a custom module in Magento 2. While modules consist of a set of classes to add new features to Magento, a theme controls how these features, and the entire website in general, will be displayed to the user. As stated in the Magento guide, a theme uses a combination of custom templates, layouts, styles, and images to provide a consistent look and feel across a Magento store.
Creating a new Magento 2 theme
We can create a theme based on a default “parent” theme or create a standalone theme from scratch. In most cases, I would recommend the first option. For this example, we will use Luma as our parent theme. The other option would be inheriting from the default “blank” theme.
Here’s an initial task list to get our new theme ready:
- Create a new directory for the theme
- Create the
registration.php
script - Create the
theme.xml
information file - Activate the new theme
Creating a new directory for the theme
While all our backend code should go in app/code
, the frontend content is expected to go in app/design
. And as our theme will only apply design changes to the frontend content, we should …
magento php ecommerce
Linux Development in Windows 10 with Docker and WSL 2
I’m first and foremost a Windows guy. But for a few years now, moving away from working mostly with .NET and into a plethora of open source technologies has given me the opportunity to change platforms and run a Linux-based system as my daily driver. Ubuntu, which I honestly love for work, has been serving me well by supporting my development workflow with languages like PHP, JavaScript and Ruby. And with the help of the excellent Visual Studio Code editor, I’ve never looked back. There’s always been an inclination in the back of my mind though, to take some time and give Windows another shot.
With the latest improvements coming to the Windows Subsystem for Linux with its second version, the new and exciting Windows Terminal, and Docker support for running containers inside WSL2, I think the time is now.
In this post, we’ll walk through the steps I took to set up a PHP development environment in Windows, running in a Ubuntu Docker container running on WSL 2, and VS Code. Let’s go.
Note: You have to be on the latest version of Windows 10 Pro (Version 2004) in order to install WSL 2 by the usual methods. If not, you’d need to be part of the Windows Insider Program to have access to …
windows linux docker containers php
Jamstack Conf Virtual 2020: Thoughts & Highlights
Welcome to Jamstack Conf Virtual 2020
Last week I attended Jamstack Conf Virtual 2020. It had originally been slated to take place in London, UK but was later transformed into a virtual event in light of the COVID-19 pandemic. The conference began at 2pm London time (thankfully I double-checked this the night before!)—6am for those of us in the Pacific Time Zone.
Up early for #jamstackconf 😎☕️ pic.twitter.com/ydjvrHWCZH
— Greg Davidson (@syncopated) May 27, 2020
Before getting too much further I wanted to mention that if you are not familiar with the Jamstack, You can read more about it at jamstack.org.
To virtually participate in the conference we used an app called Hopin. I had not heard of it before but was impressed with how well it worked. There were over 3000 attendees from 130+ countries one of the times I checked. Phil Hawksworth was the Host/MC for the event and did a great job. There were virtual spaces for the stage, sessions, expo (vendors), and networking. If you opted to, the networking feature paired you with a random attendee for a video chat. I’m not sure what I expected going into it but I thought it was fun. I met a fellow developer from the Dominican …
html css javascript conference development cdn serverless static-site-generator
Why upgrading software libraries is imperative
Image by Tolu Olubode on Unsplash
Applications primarily run on front- and back-end programming languages, including library dependencies. Operating systems and programming languages can be periodically updated to run on the latest version, but what about the many libraries being used in the app’s front and backend? As we all know, it can be quite a daunting task to maintain and individually update a long list of software dependencies like the examples later in this post. Still, it is important to keep them updated.
This post dives into our experience upgrading a complex app with a full software stack and lots of dependencies. We’ll examine the benefits of upgrading, what you will need, and how to go about such an upgrade as simply as possible.
The app in question contained decade-old software and included extensive libraries when we received it from our client. The app used languages including Java, Scala, Kotlin, and JavaScript along with many libraries. The initial plan was to upgrade the complete software stack and libraries all at once due to the gap between versions. This proved to be more difficult than expected due to a host of deprecated and removed functionality as well …
software update
Testing to defend against nginx add_header surprises
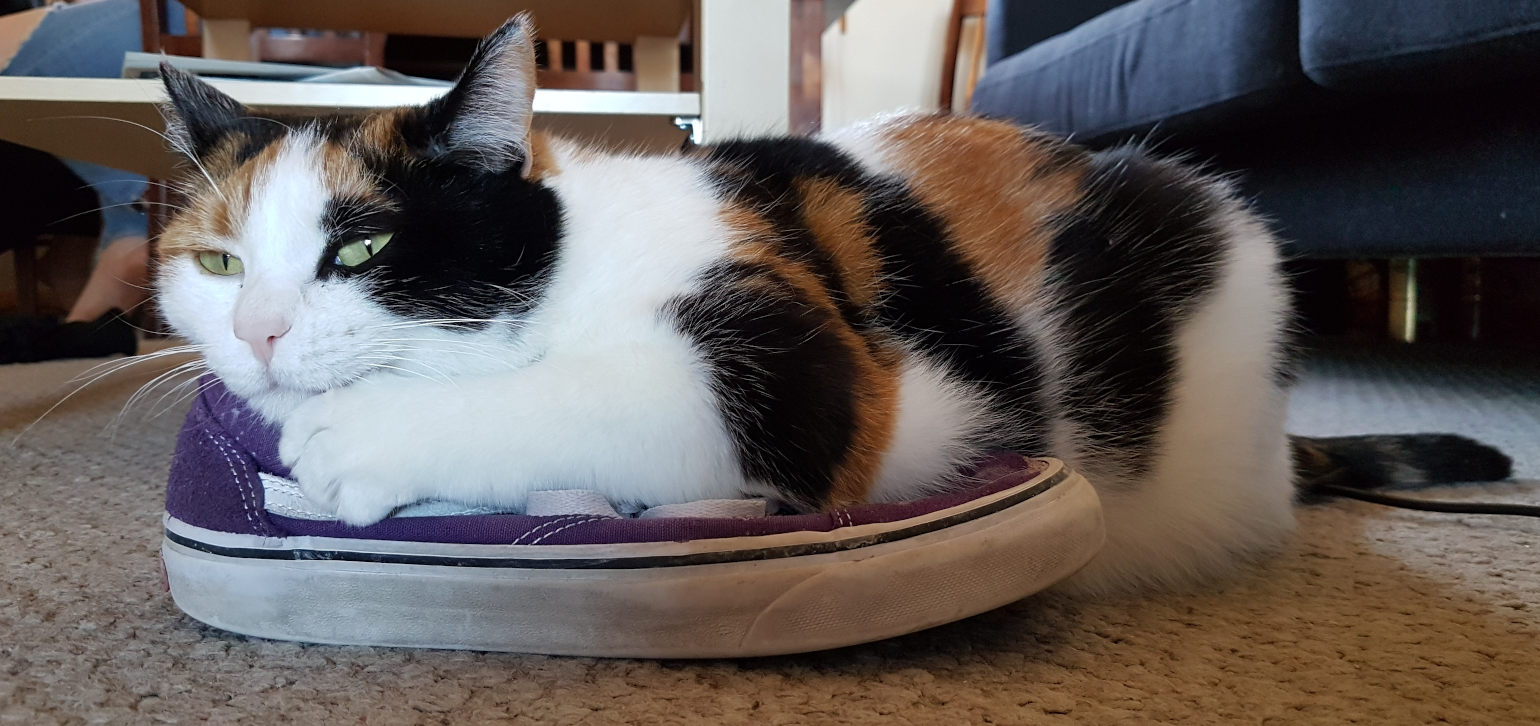
These days when hosting websites it is common to configure the web server to send several HTTP response headers with every single request for security purposes.
For example, using the nginx web server we may add these directives to our http
configuration scope to apply to everything served, or to specific server
configuration scopes to apply only to particular websites we serve:
add_header Strict-Transport-Security max-age=2592000 always;
add_header X-Content-Type-Options nosniff always;
(See HTTP Strict Transport Security and X-Content-Type-Options at MDN for details about these two particular headers.)
The surprise (problem)
Once upon a time I ran into a case where nginx usually added the expected HTTP response headers, but later appeared to be inconsistent and sometimes did not. This is distressing!
Troubleshooting leads to the (re-)discovery that add_header
directives are not always additive throughout the configuration as one would expect, and as every other server I can think of typically does.
If you define your add_header
directives in the http
block and then use an add_header
directive in a server
block, those from the http
block will disappear.
If you define …
!-->sysadmin nginx security javascript nodejs testing
Implementing SummAE neural text summarization with a denoising auto-encoder
If there’s any problem space in machine learning, with no shortage of (unlabelled) data to train on, it’s easily natural language processing (NLP).
In this article, I’d like to take on the challenge of taking a paper that came from Google Research in late 2019 and implementing it. It’s going to be a fun trip into the world of neural text summarization. We’re going to go through the basics, the coding, and then we’ll look at what the results actually are in the end.
The paper we’re going to implement here is: Peter J. Liu, Yu-An Chung, Jie Ren (2019) SummAE: Zero-Shot Abstractive Text Summarization using Length-Agnostic Auto-Encoders.
Here’s the paper’s abstract:
We propose an end-to-end neural model for zero-shot abstractive text summarization of paragraphs, and introduce a benchmark task, ROCSumm, based on ROCStories, a subset for which we collected human summaries. In this task, five-sentence stories (paragraphs) are summarized with one sentence, using human summaries only for evaluation. We show results for extractive and human baselines to demonstrate a large abstractive gap in performance. Our model, SummAE, consists of a denoising auto-encoder that embeds sentences and …
python machine-learning artificial-intelligence natural-language-processing
Designing flexible CI pipelines with Jenkins and Docker
Photo by Tian Kuan on Unsplash
When deciding on how to implement continuous integration (CI) for a new project, you are presented with lots of choices. Whatever you end up choosing, your CI needs to work for you and your team. Keeping the CI process and its mechanisms clear and concise helps everyone working on the project. The setup we are currently employing, and what I am going to showcase here, has proven to be flexible and powerful. Specifically, I’m going to highlight some of the things Jenkins and Docker do that are really helpful.
Jenkins
Jenkins provides us with all the CI functionality we need and it can be easily configured to connect to projects on GitHub and our internal GitLab. Jenkins has support for something it calls a multibranch pipeline. A Jenkins project follows a repo and builds any branch that has a Jenkinsfile
. A Jenkinsfile
configures an individual pipeline that Jenkins runs against a repo on a branch, tag or merge request (MR).
To keep it even simpler, we condense the steps that a Jenkinsfile
runs into shell scripts that live in /scripts/
at the root of the source repo to do things like test or build or deploy, such as /scripts/test.sh
. If a team member …
jenkins docker containers groovy
Creating a Messaging App Using Spring for Apache Kafka, Part 3
Photo by Pascal Debrunner on Unsplash
This article is part of a series. The GitHub repository with code examples can be found here.
In this article we’ll create the persistence and cache models and repositories. We’re also going to create our PostgreSQL database and the basic schema that we’re going to map to the persistence model.
Persistence
Database
We are going to keep the persistence model as simple as possible so we can focus on the overall functionality. Let’s first create our PostgreSQL database and schema. Here is the list of tables that we’re going to create:
- users: will hold the users who are registered to use this messaging service.
- access_token: will hold the unique authentication tokens per session. We’re not going to implement an authentication and authorization server specifically in this series but rather will generate a simple token and store it in this table.
- contacts: will hold relationships of existing users.
- messages: will hold messages sent to users.
Let’s create our tables:
CREATE TABLE kafkamessaging.users (
user_id BIGSERIAL PRIMARY KEY,
fname VARCHAR(32) NOT NULL,
lname VARCHAR(32) NOT NULL,
mobile VARCHAR(32) NOT NULL,
created_at …
java spring frameworks kafka spring-kafka-series